Generating Random Number
RPG doesn't have a random function but there are alternatives.
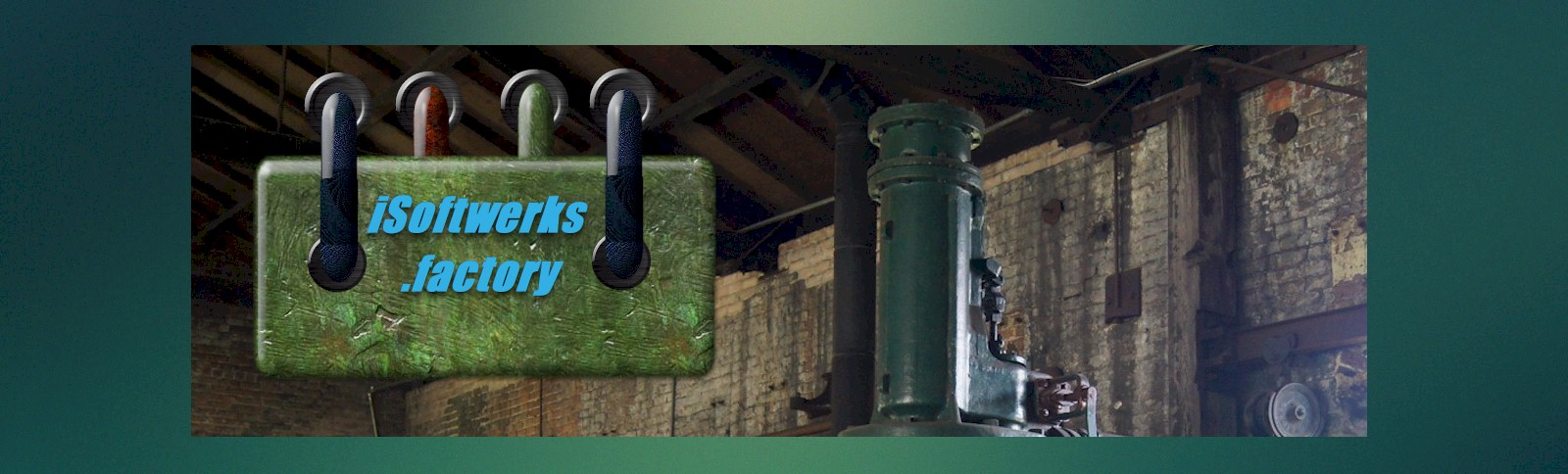
Using C to randomize
This program uses C modules listed in the QC2LE binding directory to generate random, or in this case semi-random numbers, since the first three digits of the number actually represent the day of the year. The time of day is used to seed the random number, the returned value is tested to determine if it has already been used. Binding to the C directory gives RPG some interesting functions to augment RPG op codes.H OPTION(*SRCSTMT : *NODEBUGIO) DFTACTGRP(*NO) ACTGRP('QILE') H BNDDIR('SOFTCODE':'QC2LE') **************************************************************** * PROGRAM NAME - ADM050RP * * * * FUNCTION - This program is bound to QC2LE to use the * * random number generator. * * * **************************************************************** FADMHDRPF IF E K DISK ‚ *--- ‚ /copy qrpglesrc,swcmmn_pr ‚ *--- D ADM050RP PR D OrderNbr 8s 0 D ADM050RP PI D OrderNbr 8s 0 D c0Seed S 5i 0 INZ(0) D GetRandomNbr PR 10i 0 Extproc('rand') D SetRandom PR ExtProc('srand') D Seed 10U 0 Value D randomNbr S 10i 0 D Today S 8s 0 D TimeOfDay S 6s 0 D DayNbr S 3s 0 D NewOrderNumber... D S n **-- Random Number Conversion: ---------------------------------- ** D MaxNbr S 10i 0 INZ(000099999) D MinNbr S 10i 0 INZ(000001000) ** NewOrderNumber = *off; DOU NewOrderNumber; DoU randomNbr >= MinNbr AND randomNbr <= MaxNbr; Today = DateToday(); DayNbr = DayOfYear(); TimeOfDay = TimeNow(); SetRandom(Today + TimeOfDay); randomNbr = GetRandomNbr(); ENDDO; OrderNbr = (DayNbr * 100000) + randomNbr; CHAIN OrderNbr ADMHDRPF; IF not %found; NewOrderNumber = *on; ENDIF; ENDDO; RETURN;
Using SQL to randomize
IBM does have a method for generating a random number using SQL. The SYSDUMMY1 table contains exactly one row. It is used for SQL statements in which a table reference is required, but the contents of the table are not important. The schema is SYSIBM.. . . d randomNumber s 9s 0 inz . . . exec sql select cast(rand() * 1000000000 as numeric(9,0)) as myrand into :randomNumber from sysibm/sysdummy1; . . .
The example combines the CAST function with the RAND function in a select statement to produce a nine-digit random number.