SoftCode Applications Continued
Complex Macro Instructions
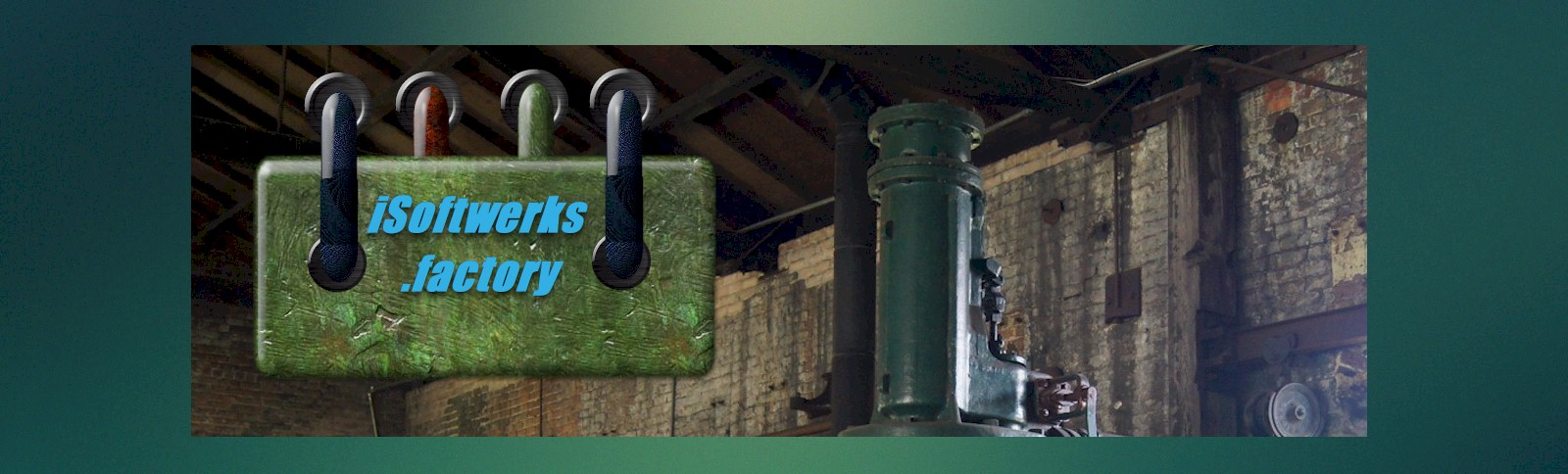
The macro instruction field of the soft coded program options and function keys is 45 bytes long. This instruction may contain several different types of operations. And in SoftCode, the macro instruction may take several different formats. An ampersand '&' in byte 1 identifies a CL command. The Soft coded function editor will pass this command on to the generic command executive program to be processed. But a more complex form of the macro can be used. (See the previous page for an external defintion of the complex macro subfields.)
000000000111111111122222222223333333334444444 123456789012345678901234567890123456789012345 <---><--------><--------><-----------------> CALL SC0195RP PLIST2 *RESERVED A | | | | | | | |___Action Code | | | | | | | | | | | |________________________________PARM list | |__________________________________________Program name |_______________________________________________Operation code 1-05 operation code 6-15 program 16-25 program data structure name 26-44 RESERVED 45-45 Action code: A = Add C = Change D = Delete V = View (display only) S = Sort Fig. G31
The following D specs contain several externally defined data structures commonly associated with the SOFTCODE development process. This subroutine is an example of using the format of the complex macro instruction to format dynamic calls based on the information provided in the macro instruction. The call becomes dynamic--the parameter list to use is populated with program variables. The prototype (see example) references a variable name instead of a literal. This means programs named on the function table or option table can be replaced with newer versions without changing the calling program. (As long as the signature hasn't changed.)
D FunctionKey E DS EXTNAME(SCKEYSPF) qualified Function keys D PGMDS ESDS EXTNAME(SCPSTSPF) Pgm status map D DSPDS E DS EXTNAME(SCDSPFPF) Display INFDS D MACDS E DS EXTNAME(SCFUNCPF) INZ Key map D OPTDS E DS EXTNAME(SCOPTNPF) INZ Option map D FUNCT E DS EXTNAME(SCMACRPF) INZ Macro map . . . D WithParms PR extpgm(SUBPGM) [1] D p$user 10 D WithParms2 PR extpgm(SUBPGM) [2] D p$user 10 D p$mode 1 D NoParms PR extpgm(SUBPGM) [3] . . . BEGSR @CALLS; EXSR @SETPM; MONITOR; SELECT; WHEN CALLPM = 'PLIST1'; CALLP WithParms(p$USER); WHEN CALLPM = 'PLIST2'; [4] CALLP WithParms2(p$USER:p$mode); OTHER; CALLP NoParms(); ENDSL; ON-ERROR; P$ERR = 'MIS0012'; ENDMON; EXSR @RETPM; ENDSR; . . . Fig. R31
The next page illustrates the function key database entries for a program named SC0320RP.